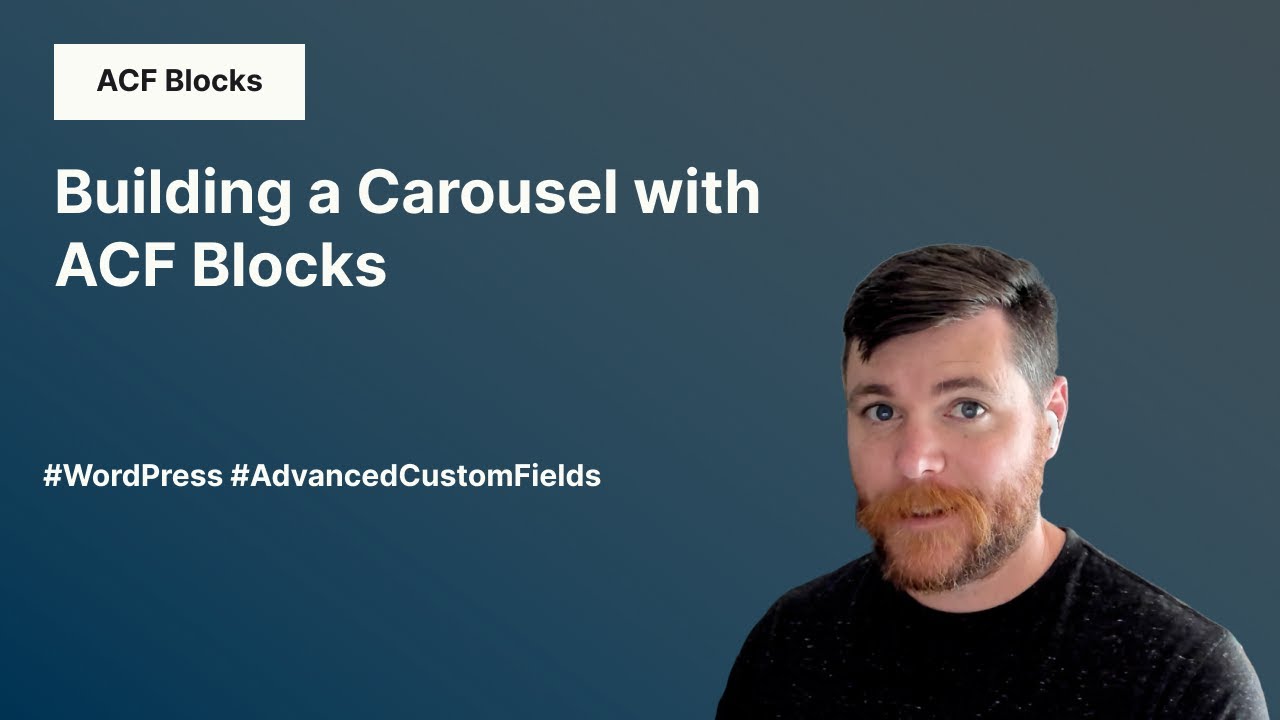
Repository: bacoords/example-acf-carousel-block
Transcript:
ACF blocks. For some people, they’re a godsend and a bridge to modern WordPress development. And for other people, they’re really tough to get right the first time. We’re gonna build a carousel block with ACF blocks, and we’re gonna walk through the process step by step. The first thing we need to do is set up our plug-in.
Our Plugin Structure
So I’ve created a plug-in here. It’s called the “Example ACF Carousel Block”. And let’s take a look at our code. You could potentially do this in your theme. A lot of times if I’m doing a theme with a lot of custom blocks, I’ll put my ACF blocks in my theme. In this case though, to make it a little more portable and to share the code, I’m doing it in a plugin.
So our plugin right now just has two files, has like the kind of auto-generated readme.txt
file and just our main plugin file with our plugin headers.
Adding a block.json
So the first thing you have to do when setting up a ACF block is you have to build the block.json
file.
This is a part where a lot of people get stopped. They kind of think they can start with the field groups or they can start with the code, but you really gotta start with that block JSON file.
So we’re gonna make a folder for our blocks. You can put your blocks anywhere. You just kind have to pay attention to where you put your blocks. I usually like to make a folder for like blocks
or something, and then a separate folder for each ACF block I’m making.
It seems like a lot at first. But if you start building a lot of blocks, it actually uh, seems to be a pretty normal and easy to use convention. So we’re gonna make a folder just for /carousel
cause we’re gonna call it the carousel block. And I’m just gonna build out my block json file.
I often will not start from scratch on a block.json
file. I’ll go to the documentation, I’ll kind of copy a starter one. So that’s what we’re gonna do here. I’m gonna copy over a block.json
file and then we are going to kind of modify it as we go. So here’s our starting block.json
file.
{
"name": "acf/carousel",
"apiVersion": "2",
"title": "Carousel",
"description": "A custom carousel block.",
"category": "formatting",
"icon": "format-gallery",
"keywords": ["carousel"],
"acf": {
"mode": "preview",
"renderTemplate": "render.php"
},
}
Code language: JSON / JSON with Comments (json)
We have the name acf/carousel
. You don’t have to use ACF. You could actually override that if you want.
Then we have the title of our block, a little description, the category and icon of where we’ll find our block, some keywords that we might add to be able to search for the block. And then this part here, the ACF key. So everything before that is standard WordPress block.json
. But once we add this acf
key, that’s basically letting it ACF know to take over and to handle the rendering in the building of the block because it’s an ACF block.
So this is not core Gutenberg, this is the extra stuff that lets us know it’s an ACF block. We really just need to pass it. Two things. We’re gonna pass it what mode we want it to look in. So we’re gonna use preview mode so you can kind of see what it looks like or you can click to edit it. And we’re gonna pass it a render template.
When you create a block, you can either render it with a function or a template. I like to use the render template where I make a separate PHP file because it’s very close to how native blocks work. And I like to do everything kind of closer to that because it’s a good practice for when you move into native blocks.
So in the same folder as our block.json
in our carousel folder, what we’re gonna do is we’re gonna make a new file and we just have to match the name, render.php
. It doesn’t really matter what you call this file. Um, it just has to match the name that you’re passing right here and just sit in the same directory.
Registering our block
The last step that we need to do before we build in our custom fields is we need to tell WordPress about our block. Um, just adding the block.json
file, turning on the plugin is not enough. We have the JSON file. We have to point WordPress to that JSON file. Let’s go back to our main plug-in file. So we’re gonna write a function, we’re gonna call it example ACF care cell block.
And we’re gonna add our function to the init action, which means it’s gonna run anytime WordPress starts. So anytime WordPress starts, our function’s gonna run, and all we need is to use register block type. Now what’s cool about register block type is this, again, is a default WordPress function. So if you were building native blocks, real WordPress blocks, this would be the same function you would use.
And we’re just gonna use it. And all we need to do is tell it where our block lives. And really we just need to tell it what directory, you know, we just wanna point it to the folder where this block lives and it’s gonna know to look for a block.json
file. And if we had more than one block, we would just repeat this line of code over and over for each of our different blocks.
So we’ll pass the directory that we’re currently in, then our blocks folder and our carousel folder. So from here we’ve added a function to the start of WordPress. We’ve told it to look for our block.json
in this folder. And so if we jump over to WordPress, we should be able to build out our custom field group in WordPress.
Adding a custom field group
We’re gonna head over to ACF field groups. We’re gonna add a new field group. It doesn’t matter what you call it, but I usually like to say block and then the name of the block that I’m building. Um, I think it makes it a little easier to look at all your field groups in the future. We’re only gonna add one field for this one, and it’s gonna be a post field.
We’re just gonna call it posts. We’re gonna leave all of the settings, except we’re gonna select multiple values cuz we’re gonna build a post carousel. So we wanna be able to pick a couple posts. Finally, we need to come down here to our location rules and assign this to our block. So we’re gonna open that dropdown and we’re gonna set the first value to block.
And then we’re gonna open the second value, and we should see our carousel block. If you don’t see your block here, that means it’s not finding your block j s o file. That means that maybe your plug-in’s not turned on. Maybe your block.json
file is not where you thought it was, or there’s some sort of mix up there.
So if you’re not seeing the name of your block, that’s the first thing to check. Is my block.json
being loaded by WordPress? Save our changes. And now we’re gonna jump over to a blog post and start using our block. So now we’re in the block editor and what we’re gonna get to do is actually use our block.
Testing our custom field
So at this point, if we return to our code, we have a block Jsun file that defined our block. We have a render.php
file, but there’s nothing actually in the render.php
file. So when we make our block, nothing is really gonna show up yet cuz we haven’t written the PHP to display our block. However, we will be able to see the custom fields.
So let’s look for our carousel. So again, nothing’s gonna show up cuz there’s nothing to render, but we see our. Custom field over here and we can switch into the edit mode here and we can see that there as well. So let’s focus on building out our render.php
file.
The render.php file
I’m gonna start by adding a little code to the top that you can actually get off of ACF’s documentation page.
And I find it super helpful cuz it kind of lays out what are all the options in this file. Okay, there we go. So this is just a big comment block, but what it’s showing us is that when we’re inside of our render.php
file, in the code of our block, these are all the variables we already have access to.
<?php
/**
* Carousel Block Template.
*
* @param array $block The block settings and attributes.
* @param string $content The block inner HTML (empty).
* @param bool $is_preview True during backend preview render.
* @param int $post_id The post ID the block is rendering content against.
* This is either the post ID currently being displayed inside a query loop,
* or the post ID of the post hosting this block.
* @param array $context The context provided to the block by the post or it's parent block.
*
* @package wpdev
*/
Code language: PHP (php)
We have the block array, which is just all of the information about the block. It’s everything from block.json
, along with all the values and custom fields that get saved. We have this content, um, that’s for an advanced feature, which we’re not gonna use at this point in time. We have, this $is_preview
, which we’re actually going to use, and what it tells us is are we in the block editor looking at a preview of the block or are we on the front end of the website?
Because we’re writing one chunk of code for both the block editor and the front end. Sometimes you’ll use that to kind of render things a little bit differently. We have the post ID of the actual post that we’re looking at. And then we have the context, which is another advanced variable we’re not gonna use in this video.
The first thing we need to do is get our custom field. Let’s head over to our block and let’s just assign some posts here so that we have some data to work with. So it’s a post carousel. So I’m gonna add in all of my example posts so that I can have, um, some examples to look at. And we’ll just hit update over here.
I’m gonna get those posts. This is my post field. I’m just gonna use Get Field Post. The great thing about ACF Blocks is I’m already inside of my block. I don’t have to do anything to get, you know, I don’t have to pass any other variables or use any other custom things. The values right there are gonna show up right here.
In fact, let’s variable jump ’em out and let’s see what it looks like. There we go. See, I can see that variable dump. It’s an array and it’s got the content of all the bulk posts. So let’s start building. Our, our front end markup. We’re gonna wrap our block in a section element and you’ll see why in a little bit, but it’s gonna kind of show us where a block starts and stops and how things are a little bit different.
So what I’m gonna do is copy in some pre-written code here, so you don’t have to watch me type it out line by line. So this here is pretty standard WordPress, php. I am checking if my post field has value. Then if so, I’m making this carousel. Um, element. Then I’m gonna loop through, set up the post data using that global post object.
global $post;
$posts_field = get_field( 'posts' );
?>
<section>
<?php if ( $posts_field ) : ?>
<div class="is-carousel">
<?php foreach ( $posts_field as $post ) : ?>
<?php setup_postdata( $post ); ?>
<div class="wp-block-acf-carousel--item">
<a href="<?php echo esc_url( get_the_permalink() ); ?>"><?php the_post_thumbnail( 'full' ); ?></a>
<h2><a href="<?php echo esc_url( get_the_permalink() ); ?>"><?php echo wp_kses_post( get_the_title() ); ?></a></h2>
</div>
<?php endforeach; ?>
</div>
<?php wp_reset_postdata(); ?>
<?php else : ?>
<p>No posts selected.</p>
<?php endif; ?>
</section>
Code language: PHP (php)
I’m going to loop out each carousel item. It’s just a post thumbnail wrapped in the permalink, and it’s a H2, the post title wrapped in a permalink. That’s gonna end my loop and reset my post data. And then later, um, if there was no post field, say the block loads empty, I’m just gonna put no posts selected so that something shows up on the back end, uh, when you first start the block.
So let’s save this and just take a look and see if it’s rendering on the front end. So let’s turn on the preview. All right, so there’s my loop, my four post example one, example two. We got our thumbnails and we got our post title, and that’s looking great. At this point, we don’t have a carousel yet because we haven’t added the any styles, any JavaScript, anything like that.
Adding a CSS Stylesheet
So right now we’re just looking at just a list of posts. So let’s add. Some custom CSS and some custom JavaScript to our block. Let’s start with our css. We’re gonna add a new style, and this is a default WordPress option that’s gonna tell. The block editor, which styles to load. So if we just wanna load kind of a local style that’s right here, we can actually just tell it file and we’ll give it a name.
We’ll just say style.css
. We don’t have any sass or a build process or anything, we just have some CSS files. So we’re just gonna add a new file to the same folder cuz it’s gonna be looking for it just like that render. It’s looking for it in the same folder. And I’m gonna put in style.css
and I’m gonna copy over just a little bit of styles for this block.
.wp-block-acf-carousel{
}
.wp-block-acf-carousel--item{
padding: 1rem;
width:calc( 100% - 2rem );
}
.wp-block-acf-carousel--item img,
.wp-block-acf-carousel--item picture{
max-width:100%;
height: auto;
}
@media screen and (min-width: 768px) {
.wp-block-acf-carousel--item{
width:calc( 33.33333% - 2rem );
}
}
Code language: CSS (css)
So here’s some basic styles. We have the carousel wrapping element, and we have no styles there. We have a few item, you know, styles what we want them to look like. Some responsive styles for larger screens, and a little bit of extra styling for the images. Let’s save our block.json
file and then we’ll go over to the block editor and see if our file got loaded.
Okay, so we’ve refreshed the page. You can see that I gave them that kind of like 33% width, and we can see that it’s working. So our blocks are loading in the styles here.
Enqueueing JavaScript
What we’re gonna do next is load up some JavaScript for our carousel. Now adding JavaScript to blocks is generally pretty painless, but with ACF blocks it can get a little tricky.
That’s because the way core Gutenberg works is it doesn’t just expect a JavaScript file. It expects. It to be part of this larger webpack build process where you have these asset PHP files that are generated and and tell you dependencies and stuff. And when you don’t have a build process like we don’t have in this plugin, you’re not gonna get a lot of that.
Like good stuff. That’s super helpful. So we have to use some of our kind of old school WordPress tricks, so we’re actually going to. Jump back to our main plug and file and we’re gonna register our scripts with WP Register script. And that way all of our JavaScript is sort of loaded, uh, the old fashioned way, and then it kind of works a lot better.
So let’s head to our main plug and file. We’re gonna add a new function here and. It’s gonna be a classic wp_enqueue_script
, where we’re just gonna do wp_register_style
and script. So the first two that we’re registering are Flickity, which is a slider library that you can use online. So we’re just loading that from a CDN right now.
I would maybe bring it into my plugin and, and copy it locally, but just for the sake of this example, we’re just loading the CSS and JavaScript for the Flickity carousel package. Then we’re gonna register our own custom script. So this script is gonna be our own JavaScript that’s gonna turn on the carousel and it’s gonna live if you see right here in our blocks/carousel/script.js
file.
So I’m gonna come in here, I’m gonna make a new file. I’m gonna call it script.js
. And I’m gonna do wp_enqueue_script
. And so what we’re doing here is we’re registering these but we’re not loading them across the entire website cuz we’re gonna let the block editor only load them on pages where we’re using the block, which is gonna be great for performance.
The other thing we have to actually do is we have to duplicate this action and do an admin_enqueue_scripts
. And what that means is we’re gonna register these on the back end of WordPress two because when you’re in the block editor, we also need access to those cause we want our preview to look just as good as the front end.
So we’re gonna save this function. We have our Flickity style, our Flickity script, and our custom script that we’re making just for our plugin that sits right here in the script js file. So let’s head back over to block.json
and we’re gonna add those here. So let’s start with our style and finish that up right here.
And we will just say, flickity. And what’s great is you can actually pass it just the name of something you registered here, and it’s gonna know how to grab it. Then we’re gonna take a script tag and we’re actually gonna do a pretty similar thing. So we’re gonna say Flickity, because that was the name of the JavaScript that we also loaded.
And then we’re gonna say, wp-block-acf-carousel
. And that’s only because that’s what I named it right here. So whatever I registered the script as, that’s what I’m going to load it up as. So I’m telling the block editor, basically, whenever you use this block, load up all of these assets. One of ’em, we can give the direct file path, but the other ones, we really have to use wp_register_script
to get ’em to work.
Initializing our carousel with JavaScript
The final thing we need to do is actually write our JavaScript that’s gonna load our carousel. So we loaded the kind of carousel library, but we need to do a little JavaScript in our custom file to initialize that carousel. So first we’re gonna make a function. We’re gonna call it initialized block.
// Helper function to initialize our carousel.
initializeBlock = function() {
const carousels = document.getElementsByClassName('is-carousel');
// Loop through all carousels.
for ( var i = 0; i < carousels.length; i++ ) {
var flkty = new Flickity( carousels[i], {
cellAlign: 'left',
contain: true,
wrapAround: true,
draggable: false,
});
}
}
Code language: JavaScript (javascript)
It’s just gonna be a function that is going to grab all of the carousels by class names. So we could use jQuery if we wanted to here, but we’re gonna try not to, because it’s always kind of nice to step away from jQuery if we can. But it’s a pretty similar approach. We’re grabbing all of the class names with is-carousel
.
And that’s because in our render, if our post field exists, the first thing we do is wrap it in this is-carousel
blog. So we’ll get them, we’ll do a basic for loop and loop through ’em and turn them all on. And what this does is instead of just initiating one, we could actually put multiple carousels on a page and it’s gonna loop through all of them and then turn them all on.
So it’s kind of a nice way to handle multiple blocks on the same page. And so this function, when we call it, we’ll turn on all the carousels, it’ll pass. These are just some basic options. You can go to the Flickity docs to kind of see what carousel options you want, if you want like icons and arrows and all that sort of stuff.
It’s all there. Um, the last thing we need to do is call this function. So when do we wanna call the function? When do we wanna initialize it? Well, it depends. Are we on the front end of the website or the back end of the website? Because again, our block is gonna load on the front end of the website and it’s gonna load on the back end of the website.
So we’re really gonna have to deal with both situations. So lemme show you how we’re gonna do that. So we’re gonna do that with this conditional, we’re gonna check if a window.acf
exists. What that means is if it exists, we’re on the backend of WordPress and ACF has loaded all of its JavaScript, and then they give us this action, which basically says, render block preview, and then the type of block, that’s our name.
if( window.acf ) {
// Initialize dynamic block preview (editor).
window.acf.addAction( 'render_block_preview/type=carousel', initializeBlock );
} else {
// Initialize on the frontend.
initializeBlock();
}
Code language: JavaScript (javascript)
We called it Carousel, right? So if we’re on the backend of WordPress and we wanna run this function to turn on all our carousel, This one is gonna work because it’s happening. It’s calling this function as part of this action that happens every time you load the block or if we’re just on the front end of the website, just call the function load our carousels.
So what’s nice about this is if I’m on the back end of WordPress and I’m slipping in and out of that preview mode, every time I go to edit my custom field, go back to the preview. This hap this runs again and it calls our function. So let’s take a look and see if everything loaded correctly or if I missed any, uh, commas or semi ones.
Okay, so I’ve refreshed my page and here we go. We can see our carousel is actually here. It’s actually clickable. I can edit it, I can mess around with it. These links are clickable. Or I can jump into that edit mode and I can rearrange the order. Maybe I want to put them backwards. Maybe I want, uh, this one here.
Maybe I want this one there. I could jump back into the preview. And boom, it’s gonna re initialize the carousel, get everything working perfectly for us. And that’s because it’s loading all of our JavaScript. It’s loading our two Flickity, uh, files that we needed, our Flickity, CSS and JavaScript, which helps us get all these little arrows and icons and everything set up.
And then it’s also loading a bunch of. Our custom, and then it’s also loading our custom JavaScript and CSS and all of that’s kind of wrapped together in the block. So let’s hit update and let’s jump over to the front end of our website and see if it’s working for us. Okay, here we go. Here’s the front end of our website.
Testing on the front end
We can see our block is loading. It has everything we need. The carousel is loading and it’s loading those assets. And if I were to go to another page on the site, it’s not gonna load any of those extra assets, which is like super performant. It’s really great for keeping our website, you know, top speed and as limited as possible.
Using Native Gutenberg Supports
So everything about this has been really great so far. But what if we wanted to take it up another level and we wanted to use some of the native Gutenberg stuff. Let’s take a look at what sort of block supports we have available to us. Let’s head into our block.JSON
file, and what we’re gonna do is we’re gonna open up the supports key.
{
"name": "acf/carousel",
"apiVersion": "2",
"title": "Carousel",
"description": "A custom carousel block.",
"category": "formatting",
"icon": "format-gallery",
"keywords": ["carousel"],
"acf": {
"mode": "preview",
"renderTemplate": "render.php"
},
"supports" : {
"align" : ["wide"],
"color": {
"text": true,
"background": true,
"link": true
}
},
"script": ["flickity", "wp-block-acf-carousel"],
"style": ["flickity", "file:./style.css"]
}
Code language: JSON / JSON with Comments (json)
Supports is a native Gutenberg feature, but we have access to it in ACF. You can check the documentation for the full list of things you can support, but we’re gonna turn on a couple of ’em. We’re gonna turn on a line and we’ll say wide. We’re gonna give the option to make our block wide if we want to.
We’re also gonna turn on some color options. We’re gonna give the option to turn on and off colors using the built-in native color palette that comes with our theme. So, When we go into the block editor, we’ll have access to all these things and then it’ll be on us to, uh, use them in our plugin. So we’re jumping back into the edit post screen.
We’re gonna click on our block here, and we’re gonna see that. Now we have this extra styles tab open because we turned on a few styles. We also have the alignment option here. So let’s test out the alignment first. Let’s go align wide and boom, it works perfect, alignwide
. Let’s go over to our colors and let’s give it a background color.
We’ll say orange. Yep. Working pretty well. And we’ll change the link color for these links right here to, how’s that pink? Uh, it’s a little hard to read. How about that? There we go. And we’re gonna update it. And so if you see, all I did was add a couple of lines of codes. My block.json
, and the color and the alignment just seems to work outta the box.
Does it work on the front end? Let’s take a look and see. So I’m back on the front end of the website and none of my cool styles showed up, right? It looks like I don’t have anything. Maybe there’s, maybe the color on one of ’em worked, but one of them didn’t. So we gotta get this working in our template.
Using get_block_wrapper_attributes
It works on the backend, but it didn’t work on our template. There’s a kind of a good reason for it, but it’s a little funky. So let’s take a look. So in our render.php
, we’re gonna use a core WordPress function that’s called get_block_wrapper_attributes
. And what get_block_wrapper_attributes
does is it takes all those settings that we just turned on, the styles, the alignment, the colors, and it’s gonna give us those as classes on our section element.
So, In the past, in ACF blocks, you would have to kind of write all of these conditionals to check for them. And sometimes you still do, depending on your theme and what settings. But for alignment and color, we can actually use this function to spit out all of those settings. Um, on our section
element, however, there is one sort of thing we do have to pay attention to here.
ACF block, it doesn’t actually work that well. Um, it really only helps us on the front end. So we’re gonna do it in a conditional, using our $is_preview
variable that we have. What we can say is, if we’re in the preview, don’t use this function at all.
If we’re in the front end of the website, um, use this function and give us all those classes that the block editor does by default. Okay, so I’ve taken. This, I’ve put, this $is_preview
. If we’re not in the preview, echo this out. If we are just echo on an empty string and, and, and move on. So we’re gonna save that.
<?php
/**
* Carousel Block Template.
*
* @param array $block The block settings and attributes.
* @param string $content The block inner HTML (empty).
* @param bool $is_preview True during backend preview render.
* @param int $post_id The post ID the block is rendering content against.
* This is either the post ID currently being displayed inside a query loop,
* or the post ID of the post hosting this block.
* @param array $context The context provided to the block by the post or it's parent block.
*
* @package wpdev
*/
global $post;
$posts_field = get_field( 'posts' );
?>
<section <?php echo ( ! $is_preview ) ? get_block_wrapper_attributes() : ''; ?>>
<?php if ( $posts_field ) : ?>
<div class="is-carousel">
<?php foreach ( $posts_field as $post ) : ?>
<?php setup_postdata( $post ); ?>
<div class="wp-block-acf-carousel--item">
<a href="<?php echo esc_url( get_the_permalink() ); ?>"><?php the_post_thumbnail( 'full' ); ?></a>
<h2><a href="<?php echo esc_url( get_the_permalink() ); ?>"><?php echo wp_kses_post( get_the_title() ); ?></a></h2>
</div>
<?php endforeach; ?>
</div>
<?php wp_reset_postdata(); ?>
<?php else : ?>
<p>No posts selected.</p>
<?php endif; ?>
</section>
Code language: PHP (php)
And what that’s gonna do is when we’re on the front end of our website, it’s actually gonna run this function here. So before I refresh the page, let’s just load up the inspectors and let’s grab our section. So here’s our section. It doesn’t really have any other classes or anything like that. We can see our content that was inside our IS carousel and all the flick atty stuff.
But um, the section element, that’s the only block. It’s sitting right at the top level. It doesn’t have any other classes or something. So what we’re gonna do is turn this on. We’re gonna save it. We’re gonna refresh now. Check out our section. Not only does it have the styles, it has the alignment, it has the color, it has, um, everything we need here.
But if you look here, it actually is spitting out all the things we need. It has the name of the block, WP Block, ACF Carousel. It has the background, it has the background color, the Align white. It does all those classes for us. So it’s super helpful to spit this stuff out automatically. I don’t have to write a bunch of code to do it, but why can’t I do it on the backend?
Um, let’s just take a quick detour and take a look why. So when we’re on the backend in the block editor with an ACF block, you’ll notice here that my section doesn’t have anything, right? Cuz we’re not spitting out all that code. It’s because there’s another diviv outside of it that ACF adds when you’re only on the backend, and this trips up a lot of people because sometimes your styles are very specifically scoped to classes and stuff, and you’re not expecting all of these classes to.
Beyond a div one level above the actual element that we wrote in our code. So it’s one sort of discrepancy between the front and the backend is this extra level of a diviv. So that’s why I find it’s kind of easiest to use the function that exists, but only on the front end and then on the backend, all of these classes, you know, all these styles that we turned on and off, it actually handles them.
Pretty easily. So it just updates the code right there. Um, and then on the front end, we’re just spitting it out on our element right here.
Recap and conclusion
So, as a quick recap, we built out our block. We wrote our block.json
file. We registered our block with WordPress. We in queued a bunch of scripts and styles for our block.
We added those back to our block.json
file. And finally we built a nice PHP template to spit out our carousel. The whole source code for this is gonna be available online. I’m hoping to do a big deeper dive into ACF and actually take each of these steps even slower and even deeper. If that sounds interesting to you, please comment and let me know, or let me know if there’s other spots of ACF that we haven’t really dug into.
Uh, and I hope this helps you tackle your first or 50th ACF block.
Full code is available here: bacoords/example-acf-carousel-block
Leave a Reply