Custom data stores in WordPress / the block editor. Hard stuff (for me at least). In this status update on the Block Styles Manager, I talk about custom REST API endpoints, handling data, and saving CSS directly to your filesystem.
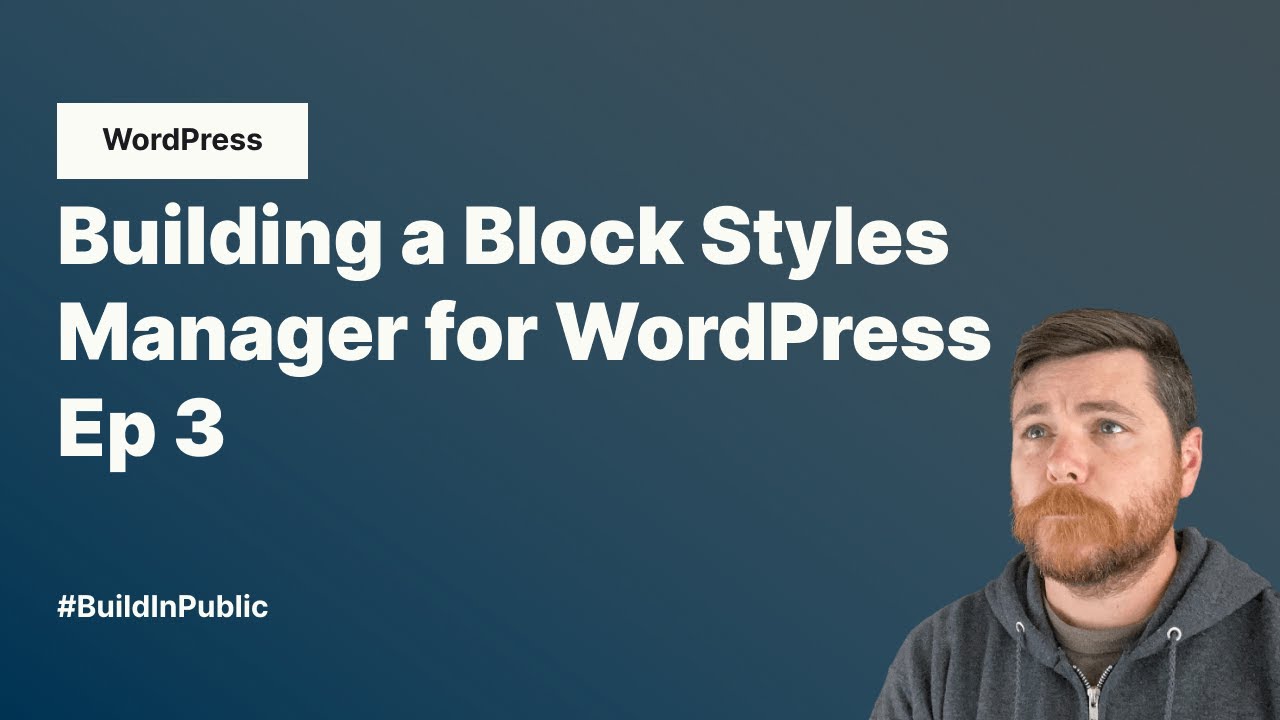
- Newsletter: https://www.briancoords.com/newsletter/
- WordPress Data: https://developer.wordpress.org/block-editor/reference-guides/packages/packages-data/
Transcript
Introduction
Welcome to part three of building the block styles manager in public. So for a little context, we’re building this block styles manager plugin that’s going to essentially let us write custom CSS in the block editor, but it’s going to do it in a way that’s a little bit more maintainable, a little bit more performant than just opening the additional CSS field or something like that.
We’re going to register styles, save them in the file system and basically, enqueue them on the front end only when they’re being used. Kind of the promise of the block editor.
The Hard Part
This was the episode I was most worried about because this is where I hit the part that I was the most afraid of. And I knew it was going to be the hardest part for this project. And I think the hardest part for anybody who’s trying to build things for the block editor, which is that I had to build my own custom data store.
So what is a custom data store and why was it so scary? Basically when I used custom post types to scaffold this data originally, what that meant was that I could use core WordPress hooks like use entity records, use entity record.
And it pulled data from WordPress and brought it into the block editor without me really having to write very much code at all. So it’s kind of the equivalent of using WP query when you’re in PHP. It’s just WordPress knows how to handle its own data. It does it really well. It brings it into
the block editor very quickly. The block editor is a giant react app. So things like data management and state management are really critical because you can’t just be API fetching every time you need something, you want a central state that kind of holds all of your data. And
that’s what a data store does. I was using the core WordPress one which I didn’t really think about it at all, but once I stepped away from using custom post types, I had to register my own data store and that’s where you kind of go from doing WordPress to basically just doing react or Redux style JavaScript.
Like it’s a very heavy JavaScript. And I bet you that for a lot of people, it’s that transition where they really say, okay, it was hard enough to even think about react.
It’s hard enough to even think about JSX. Now I have to think about data stores. It’s just really hard stuff. So I think it’s a big hurdle. I knew it was going to be a hurdle.
GitHub Copilot
And one of the things that I did while building it is I used a GitHub copilot for a lot of it. So here’s kind of an example file and at some point I will share this code, but it’s just so fast and messy.
It’s prototype code. I’ll go back later and kind of clean it up. But I want to just prove the proof of concept first. So I’m using this create Redux store function and registering a store.
It’s about keeping the data safe and not mutating and stuff, but really the other thing it does is it really just saves you a bunch of trips to the database and stuff like that.
So I had to spin up my own custom REST API endpoints. Copilot very much helped with that. Because once I said, you know, this is what I wanted to do, it kind of did it, it handled it. It was great. And then I built this store and there was a lot of times where I would do this GitHub copilot feature where I would highlight a chunk of code.
I would hit command I, which is where you open up this little copilot, and then hit the slash. There’s a couple of commands. I would do a lot of times explain, I would say, explain why this function is not returning this.
It’s doing this instead, or you know, things like that. So that ended up being really useful because normally I would have a person where I could like, kind of like bounce ideas off, but I’m kind of solo on this one. So copilot becomes like this little friend you get to talk to and just kind of say, can you explain why this is right or wrong or what I’m doing wrong here?
And it saved a lot of trips Googling, but at some point, you know, I look at this and I go, man, I feel comfortable with react. I don’t feel as comfortable with these sort of data stores. I don’t know that I’m doing it all that right. And at some point I’m going to have to sit down and find a course or something and actually learn this, this like piece of the react puzzle.
And I know it’s gonna be a lot. And that’s honestly, I think a big hurdle for a lot of people, myself included for sort of building things for the block editor and why you don’t see as many people jumping into building complicated, cool things for the block editor when you can just make your own tool, make your own page builder and stuff.
So it’s been super fun. I got it working.
Custom Data Storage
So once I was able to switch out the rest API endpoints that WordPress core provides and switch out the data storage that WordPress core provides. At that point I was actually still just pulling things from the database as custom post types, but I was basically saying, okay, I’m going to rebuild the architecture of the infrastructure in the block editor with my own custom endpoints and stuff.
Cause I know I need to. Then afterwards I did the second part, which is I made my endpoints stop using a custom post type.
So for right now, when you make a new style, a block style, and you say the name, what the class will be, what block types you want it to be available for, and you write the CSS. And again, this UI will get taken care of. It’s just not a priority. Instead of saving this in the database,
CSS File storage
I’m actually saving it as a CSS file. I’m using the WordPress convention of having a comment at the top of the file with some attributes because WordPress has a bunch of core functions that reads this information and parses it the same way that a style.
css file in your theme does this, the same way that block pattern PHP files In your theme can do this. I kind of wanted to follow that same approach, which is technically everything is saved here in the CSS file. You know, all the settings are saved up here and then it parses that when it pulls it in.
And what’s really great about this is if I make changes here and you make changes there, but this folder where we’re saving it is a version controlled Then you can just see those changes like in a really clear diff and you can see, oh look, they added the CSS and I added the CSS and you can resolve conflicts.
I’m not like exporting from the database and exporting yours from the database and getting really, like, stuck in that. There’s just no database at all. It’s just, you know, a CSS file. And honestly, my, my plugin breaks, it stops working or whatever. Here’s all my CSS sitting right there and I can just access it.
So I, I find that to be super handy and I’m setting it up with an approach where you can filter it so it’s. Right now in the WP content folder, it’ll just save all these block styles. But maybe you could put it in your theme or something like that. Sort of really modeling it a lot after ACF-JSON, that kind of local JSON approach because that’s really, I think, the best way for developers to collaborate.
Next Steps
So overall, it’s actually all working. Like you can load the block styles manager. You can write custom CSS, you can save it. It’ll say, regenerate the file for you. You could make your own file. You could add your own CSS file and it would show up in the block styles manager. So all that’s great. I think next steps, there’s a lot of things that I need to look at.
Like because I’m writing to the file system, I really need to do like a proper security check, make sure that everything that I do is really safe, secure, sanitized all that sort of stuff. So that’s the first piece of it. The next is right now I’m not really dealing with like unique classes. You know, WordPress can handle that sort of information for you.
It can make sure you don’t use the same post slug over and over, but I have to now write that. So I have to make sure we’re using unique class names or identifiers so we don’t overwrite people’s files, that sort of thing. And then, and then at the end of the day, I will move more into the UI and I think getting this a lot more cleaned up and stuff and finding a better approach cause I’m not really super happy with the modal.
I kind of want to do something that maybe. uses the sidebar more, or I’ve experimented with like a plugin, which is like how Yoast has this kind of nice sidebar. Maybe I’m not fully sure. We’ll kind of mess around because on the one hand, I like having this full view to be able to like filter and sort and stuff and find, you know, exactly what you’re looking for.
But I think once you’re like adding or editing a block style, it would be nice to be. In a little sidebar like this, where you could actually watch the code you’re rendering and that sort of thing. So, that’s my next steps, but I made it past the data store. The ability for the block editor to, you know, fetch that data from my REST API endpoints, keep it in memory and not have to refetch it every single time somebody opens one of these groups.
It can, you know write, save changes, all that stuff. So, super excited with it. Super grateful to GitHub Copilot for doing some of the tedious stuff. And we’ll see where we get with the next steps.
Leave a Reply