Extending WordPress blocks to talk to each other means using the WordPress Core Data packages, and hidden block features like “context”. With tools like useSelect and useDispatch, we’ll add a button to a core block that inserts new blocks into it’s parent block.
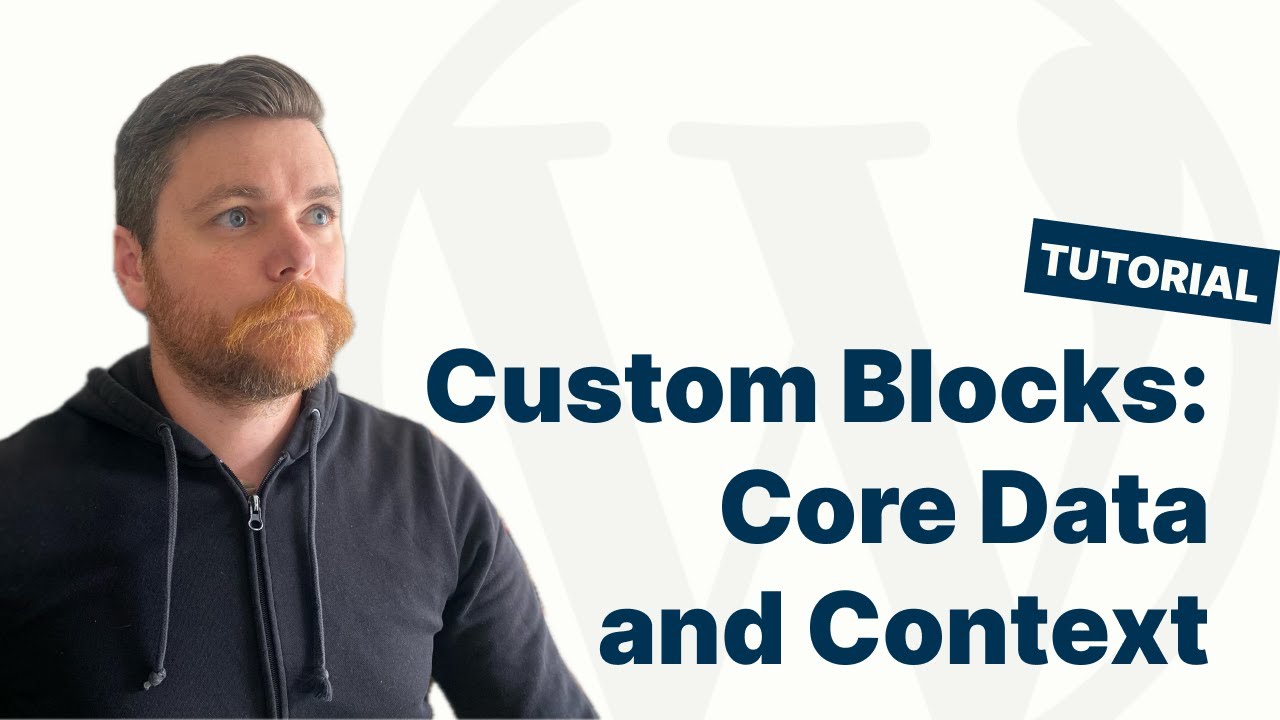
Full Transcript
Intro
Welcome. So today we’re going to talk about data in the WordPress block editor. More like, how can I take data from one block and use it in another block or communicate across multiple blocks? And the tool that we’re going to use for this is called context. So to keep in mind, the block editor is a giant react app, which means that even though you’re doing something over here, something else is happening over there.
And there’s a centralized data store. That’s kind of responsible for managing all of that data for getting content from the rest API and from your WordPress core, like your posts and your users and stuff, and showing up everywhere in the block editor, and and also handling things like the character and word count. If I update some content here, does it get updated up here? That sort of stuff. So those are the sorts of things that the block editors data storage packages are kind of always handling. And when you’re building custom block, sometimes you need to use that to trigger a change happening somewhere else.
So let’s look at what the block is that we’re modifying and what we’re building with it.
Our Block’s UI Needs
So this past weekend, I presented a very fast tutorial on building custom blocks at WordCamp Phoenix. It should be available online at some point in time for you to see. And what we built was this kind of carousel. So you can see here, I have a little carousel block on the front end of my site. And the block is actually made out of other blocks.
So the goal of this presentation was, can I make a block and just use other blocks inside of it to make it? And it was super handy. It’s actually one custom block, but inside of it is just additional media and text blocks, which sort of serve as the slides for your carousel. And so with the media and text block, you can kind of do everything where you can have some image on one side and some content on the other.
And then we use our custom block to turn that into a carousel by loading additional assets. As I was building out, there were plenty of things that I wanted to do.
The actual block itself that we built is pretty basic. It has some built in block supports like color and padding that you can use, and you can make it full or wide alignment, but that’s about it. But I thought it would be cool to add a button here in the toolbar of the block that’s basically add a slide.
Because right now the only way to add a new slide is You can have to be down here and hit this little plus icon. And if you’re like, you know, focused anywhere else, you don’t really see it. It’s kind of a pain. So I thought it would be nice to, as part of the block toolbar, add another button that said, you know, add a slide.
I also thought it would be cool if when you were in one of these media and text blocks inside, you also had an option to click that button and add a slide. And that’s where these sort of things start playing in because now what do I need to do? I need to You know, know if I’m inside of my carousel, I need to add a button.
I need that button to do something somewhere else by adding a block later in the block editor, all that sort of stuff. So it’s all possible within the block editor. It’s just there’s not great examples of how you would do this sort of thing. So that’s what we’re going to do. We’re going to first add a button here and just inject a block.
And then we’re going to do the extra step of adding a button to the media and text block. Only when it’s inside of our carousel, it can’t be just any media text block has to be in our carousel. And then I’m going to use that to inject a new slide and it’ll just make a little nice extra UI. So let’s dig into the code.
Our block scaffold
This is a custom plugin using the WordPress create block scaffold that basically built our block for us and I won’t go through how we made the inner block, but you can kind of see here that in the Block. json of our file. We didn’t really have to do too much. We just added some block supports and in the edit.
js we’re using this use inner blocks, props component to basically determine like that. We’re only allowed to have media text blocks inside and we set some custom attributes and stuff. So I’m going to that, to this video I’ll just show that, you know. A block with no other blocks inside of it is a really simple block.
There’s not a lot going on. These are pretty quick to spin up. I usually use like a template that just, I just kind of copy this and change what I want and change some attributes. And it’s a very fast way to get a block with other blocks inside of it. But we’re going to make it more complicated.
The BlockControls Component
So the first thing we need to do is we need to add block controls. Block controls are this toolbar here. So we’re going to add block controls to our block and inject a button.
Let’s import block controls from the block editor. And while we’re importing, let’s also import.
What would we need? We need toolbar group and toolbar buttons. So you can see co pilots kind of suggesting some stuff for us. So block controls is basically like a slot fill where anything we pass inside of it will show up. And then these are some components from the WordPress components package that are going to basically build out the UI for us.
So let’s come over here.
We don’t really want to break this. I love how simple this is. So we’re just going to make a little fragment and move it like that. And then we’re going to add our block controls. And so anything we put between those two tabs will basically show up on that toolbar. There’s another one you’ve probably seen called inspector controls that puts anything in the sidebar.
We want ours in the toolbar. So we’re going to add a toolbar group. And let’s set that and then we’re gonna add our toolbar button. So co-pilot’s recommending some stuff for us. Let’s yeah, let’s take it, but get rid of it. I don’t want all that. Maybe, maybe we’ll just turn co-pilot off. I feel like you’re getting in the way.
So we have toolbar group. We have our toolbar button and to our button. Actually, we’re not gonna self close. You we’re gonna. Just give you some text and say add slide, close you out, and we’re going to add an on click. So basically this is kind of like any other sort of button component, there we go.
On click insert slide. Let’s save that. Yeah, that looks pretty good. So in our block controls, we’re adding a new group of buttons. We’re adding a single button that says. Add slide. And when you click it, it’s going to run this insert slide function. And so I’m just going to define the function so it doesn’t yell at me.
But we’re not going to actually do anything. Just do that. Okay. Now the function exists. Okay. So let’s see if our button showed up.
Let’s hit our Media Text carousel and there’s our button, add slide, showing up in the toolbar of our custom block. I can see there’s a little semicolon down here, which is probably something I did in the JavaScript. Let’s find out. Yep. A little semicolon left, right there after the div.
All right. JSX. So much fun. Okay.
InsertBlock and useDispatch
So now we just need to insert the slide. This is where things get a little tricky, right? Because we need to basically, instead of something that’s inside of our block, we’re trying to make something happen outside of our block. So that’s when the core data WordPress, like data store stuff comes in handy.
This is what we’re going to do. So we’re actually going to get a function called insert block. That’s going to come out of use dispatch from the core slash block editor store. So let’s break this down. Use dispatch. Is basically a way to send information to the block editors store. So you might’ve used use select in the past, and we’re going to use that a little later on.
That’s where you select some information and dispatches where you send changes to information. And the way that, you know, data stores work is like, they’re kind of responsible for handling all of the intricacies and making sure your data here doesn’t break data there. Dispatch was going to handle making that change for us. So we just need to import use dispatch.
And that’s going to come from the WordPress slash data package. When you have GitHub co pilot turned off, that is the one thing I do miss the autocomplete on the imports, which is like 90 percent accurate. Okay. So we’re going to import use dispatch. Sometimes you have to import like a store if you have a custom store, but when you’re using a core store like this, you can just pass that.
So we’re saying, Hey, this is the store. And so inside of use dispatch, there’s a function called insert block that we get to use. Why don’t we pull up the documentation real quick to see where this comes from?
This is in the WordPress developer handbook in the reference guides and the data module reference. So there is this data module. Those are all those kinds of custom data stores and different ones. There’s different ones that are available. Some have more to do with like content on your site. So like getting like, like.
Things like that. This one has to do with the block editor itself, like the interface, the UI, like I want to make changes to things in the interface, not, you know, changes to the, you know, post content on my blog or something like that. So there’s all sorts of these things. There’s a bunch of selectors.
We’re definitely going to use some of those in a bit. And then if you go down, there’s a bunch of these actions like insert block and that’s what we’re going to do. So we’re going to. Get this insert block function from the dispatch, and then we’re going to run it to insert a block.
So this is going to happen in our insert slide. Now that we have the function available, we can run it. So if somebody clicks insert slide, we want to run insert block, and then we’re going to have to pass it a few parameters. If you go to the documentation, they will give you some parameters. I would love to see all of this turned into like a real documentation with like actual code snippets and examples and stuff, but there’s just a lot that needs to get done.
Right? So these are the parameters we need to pass it. I’m just going to show you a few things. So the first thing we have to pass it is an actual block that we want to insert. And for that, we actually have to use a function called create block.
And our create block is actually very similar to what’s happening in the template where, let me see, I don’t think I need this. Where I, you can sell like there’s some ways where there’s like name and act like some ways are different.
Not a lot of consistency, a lot of trial and error, a lot of reading documentation and finding examples. But what we’re basically saying is create a block and. Here’s the type of block and here’s the attributes. So it’s a very similar to this template function right here. And that’s the first parameter of insert block is just passing the block.
And one last thing we do have to import create block as a function.
That’s going to come from WordPress slash blocks. So there’s a lot of little pieces you have to pull, like I need to get. Stuff from the block editor for my controls. I need a function from the data that I can use to dispatch changes. I need a function from the blocks package that’ll help me create a block.
And then I need, you know, to pull out another function from that dispatch. So a lot of this stuff takes a lot of practice and a lot of research to kind of figure out, but once you do get it it does start to make a little bit more sense. So. Insert block, let’s get back to here. I’m going to make a new line just to make it a little easier to read, even though the prettier is going to take care of it for me.
So let’s go back to insert block. So we need to know an index, the number at which the block should be inserted. I’m just going to pass zero for now. So when we push this button, it’s going to insert the block at the top. It’ll be the new first slide.
And then it needs to know the root client ID. So if I don’t tell it where to insert the block, it’s actually just going to insert it, the, like into the main content, like at the top level, but if I want to insert it as a child block, then I need to pass it kind of a context. And so what I need to pass it is called the client ID.
And that’s the unique ID that’s for any block that you’re in. So every block has this unique ID in the editor. It changes. It might not always be consistent, but in this context of this editor right now. I can do that. So the block that I’m in, I’m just going to pass props. I’m going to get the client ID for the block I’m in.
And so what I’m saying is here’s my props. What I want to do is create a block, put it first and make its parent, the client ID of the block that I’m currently in. And then that should actually do the function of creating this block right here. So let’s test it out. See if I did it all right. There’s a lot of weird things to import and hopefully we got it.
Okay. So I hit my media text carousel. You can see there’s already two slides in here and if I hit add a slide, Ooh, nice. So it adds it underneath this right here has the next, you know, piece of content in, in our editor. So that’s the kind of quick, easy adding a block inside of the block that you’re already in.
Overall I would say it’s not too bad once you find the names of all of the functions. But once we figured out that we can call the insert block function, which will dispatch to the core block editor data, and we can pass it a block that we’re creating, we can say which order we want it to be in.
And we can just say where in the tree we want it to show up. Pretty simple. Only a few imports that we had to do and very simple UI with a button. So that’s the first step is going to be that. But what about the next step? What if I’m in a media and text block and I want to add a button here to this other block?
I can’t do that in my block here. I have to do that through filters. So let’s take a look at how that works.
Filtering a Core Block’s Attributes
So in order to modify the other blocks that aren’t part of this block, we’re actually going to need really some separate JavaScript, because it’s not going to be anything we do inside of this block here. So because it’s all one build process and because things kind of work together easily, what I’m going to do is I’m going to make a new file and I’m going to call it filters dot JS.
I’m going to keep it in my source folder, and I kind of like keeping it with this block because it really only is a filter I want running if my custom block is turned on. Like they kind of work together hand in hand, so I can keep it all in here. And then if I go to my index. js, that’s kind of the entry point of a custom block, that’s, this is the file that.
The build process looks at first and then it imports everything else. That’s important from here. I can just import JavaScript into here. There’s really nothing stopping me. So I’m going to import my filters to JS. And so now whatever I write in here will be pulled in as code running in the block editor.
It’ll handle all of those like dependencies and all that stuff for me. Just the same as if we’re a block. It’s just more JavaScript I get to add. So I can kind of put whatever I want in here and take advantage of the same build process.
So there’s a few problems we need to solve. And the first problem is basically how do I know that I’m this media text block inside of the carousel versus maybe let’s go over here and add a media text block down here. And this media text block is not in the carousel, right? It’s, it’s a separate thing. So that’s the first thing I need to solve is basically an awareness of when I’m Outside of the content versus inside of the block, because I don’t want to show this add slide button unless I’m inside the block.
Understanding Context
So I’m going to save that. So how do we do that? We do that through something called context. Let’s open the block. json of our custom block. So context is a, is a tool you can turn on in the block. json file, and you can basically say provides context or uses context. The easiest way to think about it is a parent block provides context, and then inner blocks inside of it can use that context and, and get passed that data automatically.
So it’s kind of like prop drilling a little bit in react. It’s just a way to pass the data. And to pass context. You have to have an attribute. The attribute is the context that you’re passing. So we’re going to add attributes. We’re actually going to add a one attribute.
So our attribute will basically say is carousel and it’ll always be, it’ll always be true.
So our is carousel attribute will exist on our parent block on our custom carousel block. And then we just need to provide it as context. So we’re going to use the provides context and you can see Copilot is still recommending things. This is actually not the way it works.
It’s actually a good example of copilot providing bad code. ’cause this is, you can see the schema is very confused. And that’s because up here I have this schema defined and VS code is smart enough to say like, I’m gonna pull in that schema and if you don’t do it right, I’m gonna let you know.
This is what I actually want it to do is I want to name my context. And then I just want to name the attribute that I’m going to pass. So this is a piece of context. That’s going to be available to some blocks and it’s going to pass the value of this attribute. And I’ve already said this attribute is a Boolean and its value is just true.
So it’s just for me a way to say. Hey, I’m passing some context that they’re, that you’re in a carousel. And so if this context exists and it’s true, then we can know that we’re inside of the media carousel block. So let’s do the counterpart, which is our carousels providing the context.
Let’s head over to our media and text block and actually use that context. So to do that, we’re going to jump into this filters. js because we didn’t write the media text block. We don’t control the block. json. We have to filter just like classic WordPress. We have to run a filter that’s going to change the block.
json, the, you know, the attributes of the media and text block on our site. So let’s start building that out.
AddFilter in JavaScript
So how do we add, how do we filter a block in WordPress? Well, the easiest, well, okay, maybe not the easiest. Okay. The most common way. And usually the way you’re going to need to go is to do it through JavaScript and not through PHP. Especially cause right now we’re in JavaScript. We’re building everything in JavaScript.
We really only need the filter when we’re in the block editor. Cause it has nothing really to do with the front end of the site. So we’re going to import, add filter from WordPress.
Books, and we’re going to add our own filter. We’re actually gonna add, I think, a couple of filters. Let’s look at how add filter works. This is in the documentation under the block filters. It’s a hook reference. So it’s coming out of that hooks package. Again, nobody ever can remember where these things come from.
But we have a bunch of different filters that you can run on, on WordPress on the block editor. So these are the block filters, and this is to modify the behavior of existing blocks. Some of these are about just like removing blocks in general. And some of these are some PHP filters. We’re going to be looking at some of these ones where you can modify.
You can modify the edit element of a block, the save element of a block, you can modify the attributes. So in this case, we’re going to be modifying let’s see, not the block attributes and gets this one, the register block type. So we’re going to modify the block as we’re registering the block. Kind of the best way to think about it is in our block, we run this register block type function, you know, and it gets all of its information.
From the block dot JSON file. And so we’re actually going to be kind of filtering a lot of that metadata information when the block gets registered. Let’s start here. Let’s put in that filter that we just looked at blocks dot register block type. And sometimes you can hover over. I can hover over some of these and I can kind of get some, you know, it’s nowhere near what it was like in the WordPress PHP world where you get like the full code doc block and stuff.
I actually don’t know if that’s something I can turn on. If you do know, put it in the comments, please, but you get kind of some basic stuff. So the next one is my namespace. So I’m just prefixing this WP dev WordCamp Phoenix 2024, because that’s the repo that I’m in that I’m working through and then a function.
And the function is going to give me. Two things. It’s going to give me the actual settings that we get to modify. And I just think of it as like what you see in the block. json. There’s other stuff, but I think of it mostly as that. And then the name of the block and that name of the block is super handy because what we can do is we can say if core slash media text does not equal the name, just return the settings.
And it’s important to note that this is a filter, not an action, right? So just like in WordPress, PHP filters, you have to return the value, otherwise you kind of break everything. So what we’re saying is if we’re not in a media text block just return the settings because what we’re trying to modify is the media text block.
But if we are in the media text block, let’s return the settings, but let’s override and add uses context with. WP dev, WordCamp Phoenix,
- So uses context here, essentially matching what we did here, right? Well, this is the context that we provided. Now we’re saying, Hey, make sure you use it. And honestly, I should probably be doing this in case there is anything else there. Like that. There we go. That’s. The kind of safe way. I don’t want to ruin anybody else’s use this context.
Okay. So there’s our filter. This one’s pretty simple. It’s not going to do anything like on the front end. All it’s really doing is basically saying that now the media text block gets this additional context. We could kind of do like some console logging and see like it in the command line, but I think we’re okay on that.
Just refresh and make sure nothing is breaking. We’re not ruining anything here. Okay, perfect. So that’s our first filter. We’re just saying, Hey, core media tech spot, you know, have some new context that you can use. That’s pretty simple. Let’s get to the complicated part, which is using that context.
Modifying a core block’s Edit.js
But now it’s time to actually add some UI to the media text block with that add slide button. And so this is where we get to really play with how did I get that context? How did I use it? How do I inject blocks somewhere else, you know, over here in the block editor, that sort of thing. So this one’s gonna be kind of a lot, but let’s dig through it.
We’re going to just start with an edit function and
Think of the edit function as just as if you were building a custom block and in your custom block, you have this edit right here. We’re basically adding to this or kind of like a running alongside of it. So let’s add the edit function with the filter, and then we’ll come back and actually like put the stuff in it.
But let’s just talk about how we even get it to go. So the same way we’re going to do an add filter where, you know, again, we’re filtering something in the block editor, but
this time we’re filtering the block edit component. We’re using this editor dot block at it. So this one actually does have a pretty decent little code example here. And so they have actually some interesting things like they mentioned, Hey, you know, this hook is constantly running. So you know, there’s some things you can do to kind of make it a little bit more streamlined.
Because you know, you don’t want this, this will basically run on every block unless you kind of control it a little bit. So this is what we’re going to do. Let’s go through that.
So our namespace again and then this is where you need to pass it a react component. And yes, we do. We are making our little like react component here, but we actually need to combine two components together. So you end up having to pass it this function called create higher order component and it gets the block edit value.
And then we’re going to. Do a little return and it’s actually going to return a function that passes us the prop. So this is where you get a little complicated. You’re like return inside of a return, making sure we have everything here. But basically what we’re going to do is we’re going to make our react component.
We’re going to get access to this block edit that we’re filtering. That’s going to return a function similar to just like we were here, similar here, like you’re returning a function with some props in it. So we’re just kind of passing all of that through. And then from here we can do what it was saying, which is, you know, make sure that you’re not running this everywhere.
So it’s very similar to what we did here. We’re just going to check.
We’re basically going to say, Hey, if we’re not in the core media text block, return just the component. And again, it’s a filter, so we have to return the component. We can’t just return nothing. We have to return that. And we’re going to just pass its props back to it.
Brackets there. So we had our props, we’re going to pass back the props and just return it. So this is basically saying like we were given a block edit, we took out its props, we’re sending it all back to you if it’s not the core media text block. Hey, now, if it is, then we’re going to return the same thing. So the same block edit component that was there, but. We’re going to also return our own edit component. So we’re returning two components and then we just need these props. So we’re saying when you build out the edit component that, you know, of the block in the block editor, also right next to it with all the same props and context and everything, run my little function here and render that as well and let that exist.
So. It’s basically our way of kind of injecting stuff into other blocks in the block editor. And, you know, from there, now it’s just a matter of actually building out our edit component and grabbing the context, making the changes, injecting the block, adding the button, all that sort of stuff. So let’s work on that.
And I guess one last thing I kind of forgot is I do need to import this as well.
Just to make sure that’s running. Okay.
Adding UI a core block’s toolbar
So we’re in our edit function. We’re imagining, you know, conditionally we’re inside the media and text block. We’re going to do a quick check first for our context. So if you remember, we. Added this context right here. And we know it’s going to return true if they’re inside the carousel, because we set that attribute as a Boolean and we set it to always be true.
And if they’re not, then they won’t get the context or it’ll be a falsy value.
So let’s just say if no props. context.
Then we can return null. Basically, you know, if this context is not being passed down or it’s coming back as false in either situation, just return null and it’ll just be our normal block edit and this’ll just be nothing. Nothing will happen. The rest of this code won’t run. It’ll only run if we’re inside that media text block.
So we need a few things. Let’s go back here. We definitely need this and we’re going to need this. Although it’s not going to look the same, but we’ll start with it. We know that we want to do these two things. We know we want to insert the block and we know that we want to insert the new slide, but we’re going to need a little bit more than that.
So let’s come up here. We need these. Toolbar group, right? Cause we’re going to put in some block controls. Yeah. So we need all this stuff. Let’s just put them in now to save ourselves some time. We’re going to need that block controls, remember that, that slot where we get to pass in toolbar stuff.
So let’s get, do that, get rid of that, get rid of that. Okay. We’re going to need all that stuff for sure. So we can start putting that stuff in. We know that we are going to need this. We know that we need to do all this. This is where we’re going to get in trouble. We don’t, we need, we’re going to need the client ID.
So. Let’s build out, let’s copy a few more things. Let’s just come over and let’s just copy this because we know it’s the same thing. So in here we’re going to return that. So this is returning just the UI of the component. And it’s going to be the same exact thing. It’s going to be that block toolbar, it’s going to be a button that says, add slide.
When you click it, we insert the slide, just like we built before. It’s going to do all the same stuff. This is where we need to kind of move things around because this is not going to work. Because before, you know, where do we want to insert the block? We want to insert it inside of the Media Text carousel, but in order to put it inside the Media Text carousel, we need the client ID of the Media Text carousel, which we had last time, but we don’t have this time.
So we actually need to grab that, that ID. There’s a few ways to handle it. You can, I believe. Pass it through the props. That’s one way. So like we passed it through, like how we pass this context. Some people will save the client ID there and pass it down. That’s one way I thought it’d be more interesting to show what it’s like to pull it out of the block editors data storage instead, because it’s just kind of an example to get to see how that works.
Using useSelect to get Block Data
So we’re going to keep this the same. We’re, we need our insert block function, but what we actually still need is we need our parent block. So how do we get our parent block? This is where use select comes in handy. So you select is that counterpart to use dispatch. So. Where I imported, use dispatch. I can also import you select and remember dispatches to send changes, but select is to get information.
So one sends information, one gets information that’s ridiculously simplistic, but I think it gets the job done. So you select, usually looks like this. You have a function and you get something called select passed into it. It’s what’s called a hook . It’s a very nice way to get information and it runs once it’s kind of like that use effect thing where It gets the data when it needs it it updates it when it needs it but it doesn’t continually keep fetching every single time you render because it’s a like a use kind of hook. You might see old block editor tutorials that show things like with select and that sort of stuff Not as handy because those were just earlier iterations and now This sort of approach is getting really good So What we have here is we want to get the parent block.
We basically need to return the parent block here. So let’s, let’s make parent block a variable and we’re going to like solve it. So we have access to select cause that was just passed to us. And similarly, we’re going to use the same data store. So before we were using the core block editor, we’re going to use the same one cause that’s the data store that has information about all the blocks.
So we’re going to do that. And then it has an additional function on it. And let’s jump into the docs real quick.
Let’s jump back to the block editor data. This is where we sort of had our create block and all that sort of stuff. So we’re looking for something called get block parents by block name. So there’s all sorts of these weird ones. It feels like they just kind of make them up when they need them or something like.
Get block root client ID sounds like it would be good, but then if our carousel is not the root, like if it’s inside of a group or in a column or something, then it’s not technically the root. Like it’s all these weird things. So get block parents by block name seems to be the function that works the best for this situation, because if you give it a block client ID and you name a specific type of block, it’ll go and order up its parents and.
For any block that matches that, and you can grab the first, second, third one. So how does this work in practice? We’re going to run, get parents block by parents name. And at first we have to pass it the client ID. That’s, you know, the unique ID for the block we’re in, which we’re getting for our block from the props, we’re going to pass it that.
And then we need to know what block is it looking for. So like before we were looking for like core media text block, we actually want this block here, which is this kind of same filter name thing that I’ve been using everywhere, kind of easy to handle. So we’re basically saying. Start at this block, go up through its parents until you find one of these and pass it to us.
And then it’s going to pass you just like a client ID, but we’re actually going to do is we’re going to run select again with that same core editor. So this one is going to just return the client ID actually with the whole block. Yeah, so it returns an array of client IDs of all the parents that match this particular parameter.
I feel like I’m, I feel like I’m jQuery land with this kind of stuff. Okay. And we’re going to use a second function that comes out of this select called get block. And
we’re basically going to say is get the parent block, get the first one out of the array. I mean, I could do some more sanity, like make sure to like the parent block return something and make sure the first one exists, but like at the end of the day, I think it’s fine. We’re, we’re basically getting the parent blocks client ID.
I actually want the whole block, not just the client ID. So I’m calling another function from that same thing. And get block. Here, because now that I have its client ID, I can get the full block. And then I’m going to return parent block
and that parent block is going to get passed to this here. Okay. Now we have our parent block. So this honestly used to take like 30 lines of code. Now it’s kind of done in like. Six or seven lines of code. It’s getting a lot better. The more that they add these like random you know, function names the easier it is for all of us.
Okay. So we’re going to do the same thing. We’re going to create a block. This second piece was was the order, right? So what I’m going to do is I’m going to grab my parent block. I’m going to hit inner blocks and I’m going to do the link. And then I just need the parent block dot client ID. Yeah, so now I’m saying when you insert a block, insert it inside of that parent block and Because I know how many slides how many inner blocks it has I can actually have it inserted at the end You could get fancy and try to figure out where you are and insert it directly after the current block But what I’m doing is basically if I leave this at zero, it’ll insert it at the beginning If I give it the length of the total number of inner blocks, it’ll insert it at that position Okay, so that should handle everything because if they click the button, we call this function and this function has access to parent block, which we already got when the block initiated and everything we’ve imported everything.
We should be good to go. Let’s test it out and see if it works.
and we have one error, underscore, underscore is not defined. Okay. Let’s just copy it from here to our translation function, which I forgot to import just to get right there. Let’s try again and see if our block works.
So we’re in our media text carousel. We have that slide. We go to our media text one inside the carousel, and now you can see we have a new add slide button added to it. And if we go to our media text carousel. Not our media text block, not inside of our carousel, but this separate one. In fact, let’s just add like a space or before.
So we can like really see this one is way outside. Does not get the add slide button because it did not get the proper context. So it didn’t. It just dropped out our media text blocks inside of our carousel did get the context now click it Nope, and it works. It adds it to the end. There we go And our new one has one hope and we can keep doing it and if we’re select our parent We can do it there, too This one adds it at the front the internal ones out at the end
Next Steps
Definitely some room there to improve that experience a lot more that we could do with this.
I think You know There could be settings for, so it’s a carousel, right? There could be settings to control all of this stuff that we could build into our block. There’s so many ways to adapt it, but I just thought the block was sitting there. It was kind of a fun one to do for WordCamp Phoenix, and it would be a great opportunity to show how I would extend it.
And everything that we needed to do was either. In the block itself just with a little extra toolbar control right there, or in this filters file that we’re just importing and running and we’re kind of making some modifications. And so that’s it. So context, great way to modify blocks. Great way to pass data between blocks.
The data store package with use select and use dispatch are great ways to trigger changes in the block editor, make other changes, remove, insert things, that sort of stuff. And. While the stuff is not as well documented as I would like, I think it’s starting to get super powerful and often it’s not a matter of, can I do this in the block editor?
It’s starting to become a matter of just how do I do this in the block editor, which is a, at least an improvement, a better place to be. So leave me a comment if you have any questions, anything you’d like to see. Maybe I’ll go further on this block and add some settings and pass them through JavaScript or something fun like that.
Or if you have any other ideas you’d like to see. And be sure to subscribe for future updates and more block editor development tutorials.
Leave a Reply